Shopify Hydrogen: build a fully functional price range filter component
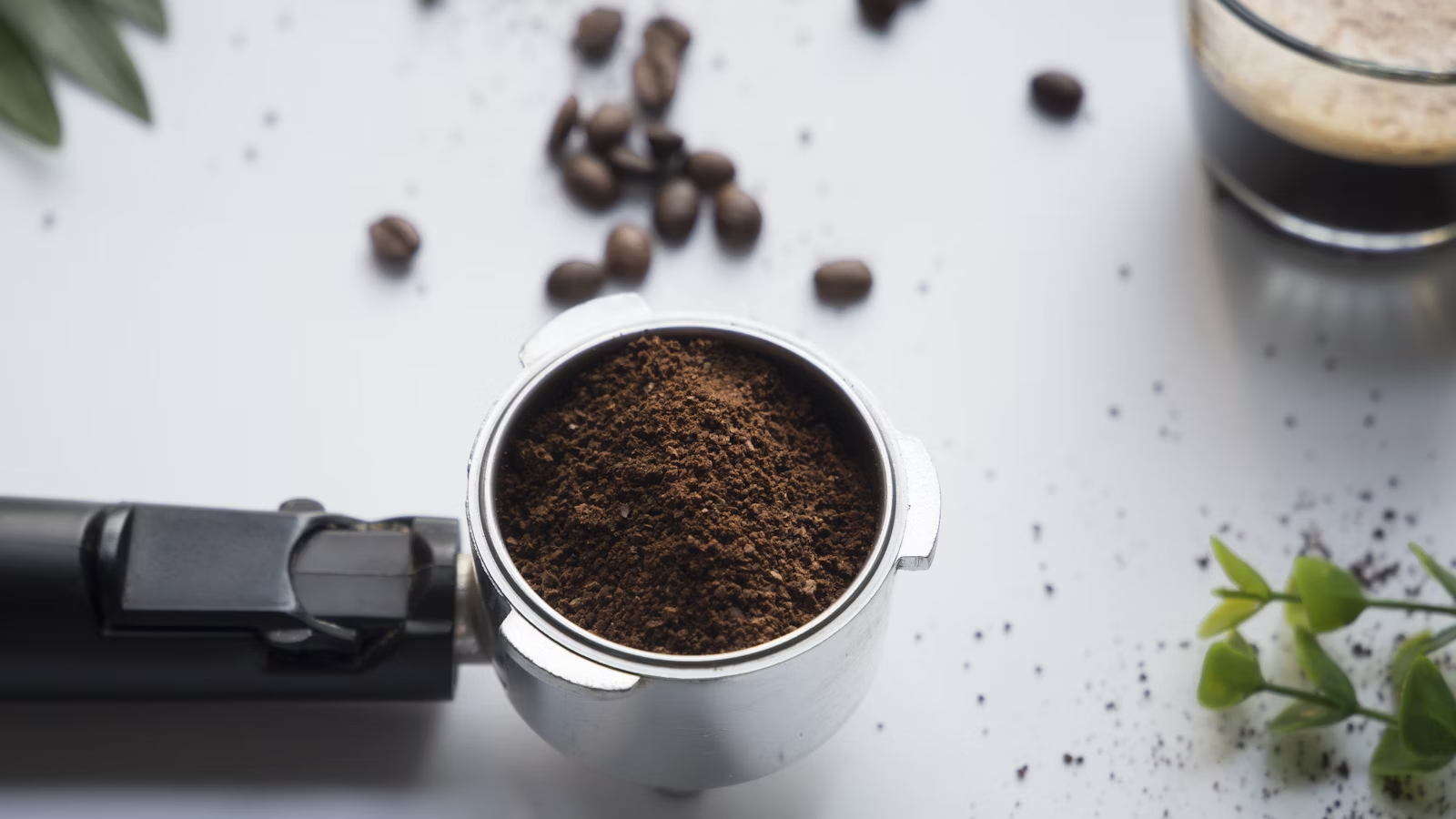
- Published on
- /8 mins read/---
A price range filter component is a common feature in e-commerce websites. It allows users to filter products by price range, and often be used in collection pages.
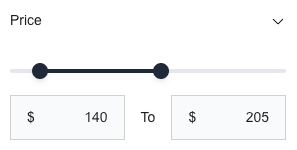
In this article, I will show you how to build a fully functional price range filter component for a Shopify Hydrogen storefront.
Querying products and parsing price filters
In your collection route loader function, you will need to query the products and parse the price filters from the query parameters.
Get all filters from query parameters
All filters are stored in the request query parameters, and should have the same filter prefix to differentiate them from other params.
import type { LoaderFunctionArgs } from "@shopify/remix-oxygen"
import type { ProductFilter } from "@shopify/hydrogen/storefront-api-types"
const FILTER_URL_PREFIX = "filter."
export async function loader({ request }: LoaderFunctionArgs) {
let { searchParams } = new URL(request.url)
let filters = [...searchParams.entries()].reduce((ft, [k, v]) => {
if (k.startsWith(FILTER_URL_PREFIX)) {
ft.push({
[k.substring(FILTER_URL_PREFIX.length)]: JSON.parse(v),
})
}
return filters
}, [] as ProductFilter[])
// ...
}
Query products with parsed filters
After parsing the filters, we will pass them to the collection query to get the products.
import type { LoaderFunctionArgs } from "@shopify/remix-oxygen"
import type { CollectionQuery } from "storefront-api.generated"
export async function loader({ params, context }: LoaderFunctionArgs) {
let { collection, collections } = await context.storefront
.query<CollectionQuery>(COLLECTION_QUERY, {
variables: {
filters,
handle: params.collectionHandle,
// more variables including pagination, sort, language, country
},
})
.catch(() => {
return { collection: null, collections: [] }
})
}
The COLLECTION_QUERY
should also include the lowest and highest price products to be used for the price range filter.
const COLLECTION_QUERY = `#graphql
query collection(
$handle: String!
$filters: [ProductFilter!]
// ... more variables
) {
collection(handle: $handle) {
id
handle
title
products(
filters: $filters,
// ... more variables
) {
filters {
id
label
type
values {
id
label
count
input
}
}
// ... more fields
}
highestPriceProduct: products(first: 1, sortKey: PRICE, reverse: true) {
nodes {
priceRange {
minVariantPrice {
amount
currencyCode
}
maxVariantPrice {
amount
currencyCode
}
}
// ... more fields
}
}
lowestPriceProduct: products(first: 1, sortKey: PRICE) {
nodes {
priceRange {
minVariantPrice {
amount
currencyCode
}
maxVariantPrice {
amount
currencyCode
}
}
// ... more fields
}
}
}
// ... more fields
}
` as const
Extracting applied filters and prettifying the price filter label
Some filters are not having any values, so we need process the params to extract the applied filters and prettify the price filter label.
import type { I18nBase } from '@shopify/hydrogen'
import type { ProductFilter, CurrencyCode } from "@shopify/hydrogen/storefront-api-types"
let allFilterValues = collection.products.filters.flatMap(
(filter) => filter.values,
)
let appliedFilters = filters
.map((filter) => {
let foundValue = allFilterValues.find((value) => {
let valueInput = JSON.parse(value.input as string) as ProductFilter
// special case for price, the user can enter something freeform (still a number, though)
// that may not make sense for the locale/currency.
// Basically just check if the price filter is applied at all.
if (valueInput.price && filter.price) {
return true
}
return (
// This comparison should be okay as long as we're not manipulating the input we
// get from the API before using it as a URL param.
JSON.stringify(valueInput) === JSON.stringify(filter)
)
})
if (!foundValue) {
console.error("Could not find filter value for filter", filter)
return null
}
if (foundValue.id === "filter.v.price") {
// Special case for price, we want to show the min and max values as the label.
let input = JSON.parse(foundValue.input as string) as ProductFilter
let min = parseAsCurrency(input.price?.min ?? 0, locale)
let max = input.price?.max
? parseAsCurrency(input.price.max, locale)
: ""
let label = min && max ? `${min} - ${max}` : "Price"
return { filter, label, }
}
return { filter, label: foundValue.label }
})
.filter((filter): filter is NonNullable<typeof filter> => filter !== null)
// The helper function to parse the price as currency.
function parseAsCurrency(
value: number,
locale: I18nBase & {
label: string
currency: CurrencyCode
pathPrefix: string
}) {
return new Intl.NumberFormat(`${locale.language}-${locale.country}`, {
style: "currency",
currency: locale.currency,
}).format(value)
}
That's all the data needed for the price range filter component. Send them to the client by returning in the end of the loader function.
import type { LoaderFunctionArgs } from "@shopify/remix-oxygen"
export async function loader({ params, context }: LoaderFunctionArgs) {
// ... loader function logics
return json({
collection,
appliedFilters,
// ... more data
})
}
Building the price range filter component
Since Hydrogen is built on top of Remix, this component will simply be a React component.
I will use Radix UI
for the slider component, and Tailwind CSS for the styling.
import * as Slider from "@radix-ui/react-slider"
import { useLocation, useNavigate, useSearchParams } from "@remix-run/react"
import clsx from "clsx"
import { useRef, useState } from "react"
import type { CollectionQuery } from "storefront-api.generated"
const FILTER_URL_PREFIX = "filter."
export function PriceRangeFilter({
collection,
}: {
collection: CollectionQuery["collection"]
}) {
let [params] = useSearchParams()
let location = useLocation()
let navigate = useNavigate()
let thumbRef = useRef<"from" | "to" | null>(null)
let { minVariantPrice, maxVariantPrice } = getPricesRange(collection)
let { min, max } = getPricesFromFilter(params)
let [minPrice, setMinPrice] = useState(min)
let [maxPrice, setMaxPrice] = useState(max)
function handleFilter() {
let paramsClone = new URLSearchParams(params)
if (minPrice === undefined && maxPrice === undefined) {
// Remove the price filter from the URL if both min and max are undefined.
paramsClone.delete(`${FILTER_URL_PREFIX}price`)
} else {
// Safely update the price filter.
let price = {
...(minPrice === undefined ? {} : { min: minPrice }),
...(maxPrice === undefined ? {} : { max: maxPrice }),
}
paramsClone = filterInputToParams({ price }, paramsClone)
}
if (params.toString() !== paramsClone.toString()) {
// Navigate to the new URL with the updated filters.
navigate(`${location.pathname}?${paramsClone.toString()}`, {
preventScrollReset: true,
})
}
}
return (
<Slider.Root
min={minVariantPrice}
max={maxVariantPrice}
step={1}
minStepsBetweenThumbs={1}
value={[minPrice || minVariantPrice, maxPrice || maxVariantPrice]}
onValueChange={([newMin, newMax]) => {
if (thumbRef.current) {
// Prevent the min thumb from being dragged to the right of the max thumb.
// And prevent the max thumb from being dragged to the left of the min thumb.
if (thumbRef.current === "from") {
if (maxPrice === undefined || newMin < maxPrice) {
setMinPrice(newMin)
}
} else {
if (minPrice === undefined || newMax > minPrice) {
setMaxPrice(newMax)
}
}
} else {
setMinPrice(newMin)
setMaxPrice(newMax)
}
}}
onValueCommit={handleFilter}
className="relative flex h-4 w-full items-center"
>
<Slider.Track className="relative h-1 grow rounded-full bg-gray-200">
<Slider.Range className="absolute h-full rounded-full bg-gray-800" />
</Slider.Track>
{["from", "to"].map((s: "from" | "to") => (
<Slider.Thumb
key={s}
onPointerUp={() => (thumbRef.current = null)}
onPointerDown={() => (thumbRef.current = s)}
className={clsx(
"block h-4 w-4 bg-gray-800 cursor-grab rounded-full shadow-md",
"focus-visible:outline-hidden",
)}
/>
))}
</Slider.Root>
)
}
The utils needed for the price range filter component are:
import type { ProductFilter } from "@shopify/hydrogen/storefront-api-types"
import type { CollectionQuery } from "storefront-api.generated"
function filterInputToParams(
input: string | ProductFilter,
params: URLSearchParams,
) {
let filter =
typeof input === "string" ? (JSON.parse(input) as ProductFilter) : input
for (let [k, v] of Object.entries(filter)) {
let key = `${FILTER_URL_PREFIX}${k}`
let value = JSON.stringify(v)
if (params.has(key, value)) {
return params
}
if (k === "price") {
// For price, we want to overwrite
params.set(key, value)
} else {
params.append(key, value)
}
}
return params
}
function getPricesRange(collection: CollectionQuery["collection"]) {
let { highestPriceProduct, lowestPriceProduct } = collection
let minVariantPrice =
lowestPriceProduct.nodes[0]?.priceRange?.minVariantPrice
let maxVariantPrice =
highestPriceProduct.nodes[0]?.priceRange?.maxVariantPrice
return {
minVariantPrice: Number(minVariantPrice?.amount) || 0,
maxVariantPrice: Number(maxVariantPrice?.amount) || 1000,
}
}
function getPricesFromFilter(params: URLSearchParams) {
let priceFilter = params.get(`${FILTER_URL_PREFIX}price`)
let price = priceFilter
? (JSON.parse(priceFilter) as ProductFilter["price"])
: undefined
let min = Number.isNaN(Number(price?.min)) ? undefined : Number(price?.min)
let max = Number.isNaN(Number(price?.max)) ? undefined : Number(price?.max)
return { min, max }
}
You might include 2 inputs for the min
and max
price to make it more user-friendly. The 2 inputs values will be synced with the slider.
export function PriceRangeFilter({
collection,
}: {
collection: CollectionQuery["collection"]
}) {
return (
<div className="space-y-4">
<Slider.Root>
// Slider component
</Slider.Root>
<div className="flex items-center gap-4">
<div className="flex items-center gap-1 px-4 border border-line-subtle bg-gray-50 shrink">
<VisuallyHidden.Root asChild>
<label htmlFor="minPrice" aria-label="Min price">
Min price
</label>
</VisuallyHidden.Root>
<span>$</span>
<input
name="minPrice"
type="number"
value={minPrice ?? ""}
min={minVariantPrice}
placeholder={minVariantPrice.toString()}
onChange={(e) => {
let { value } = e.target;
let newMinPrice = Number.isNaN(Number.parseFloat(value))
? undefined
: Number.parseFloat(value);
setMinPrice(newMinPrice);
}}
onBlur={handleFilter}
className="text-right focus-visible:outline-hidden py-3 bg-transparent w-full"
/>
</div>
<span>To</span>
<div className="flex items-center gap-1 px-4 border border-line-subtle bg-gray-50">
<VisuallyHidden.Root asChild>
<label htmlFor="maxPrice" aria-label="Max price">
Max price
</label>
</VisuallyHidden.Root>
<span>$</span>
<input
name="maxPrice"
type="number"
value={maxPrice ?? ""}
max={maxVariantPrice}
placeholder={maxVariantPrice.toString()}
onChange={(e) => {
let { value } = e.target;
let newMaxPrice = Number.isNaN(Number.parseFloat(value))
? undefined
: Number.parseFloat(value);
setMaxPrice(newMaxPrice);
}}
onBlur={handleFilter}
className="text-right focus-visible:outline-hidden py-3 bg-transparent w-full"
/>
</div>
</div>
</div>
)
}
That's how we build a fully functional price range filter component for collection pages in Shopify Hydrogen.
Check out the demo video below:
References
The component is fully open-sourced and available on GitHub.
Bonus
I'm building the first-ever Shopify Hydrogen theme customizer and CMS, including multiple Hydrogen Themes (all are free and open-sourced). If you are interested in building your own Shopify Hydrogen online store, checkout these links:
Happy filtering .